Figure at the end of this page shows the meta model for Java executable code.
There are two main kinds of code elements.
First, statements CtStatement
(javadoc)
are untyped top-level instructions that can be used directly in a block of code.
Second, expressions CtExpression
(javadoc)
are used inside the statements. For instance, a CtLoop
(javadoc)
(which is a statement) points to CtExpression
which expresses its boolean condition.
Some code elements such as invocations and assignments are both statements
and expressions (multiple inheritance links). Concretely, this is translated as an
interface CtInvocation
(javadoc)
inheriting from both interfaces CtStatement
and CtExpression
.
The generic type of CtExpression
is used to add static type-checking when transforming programs.
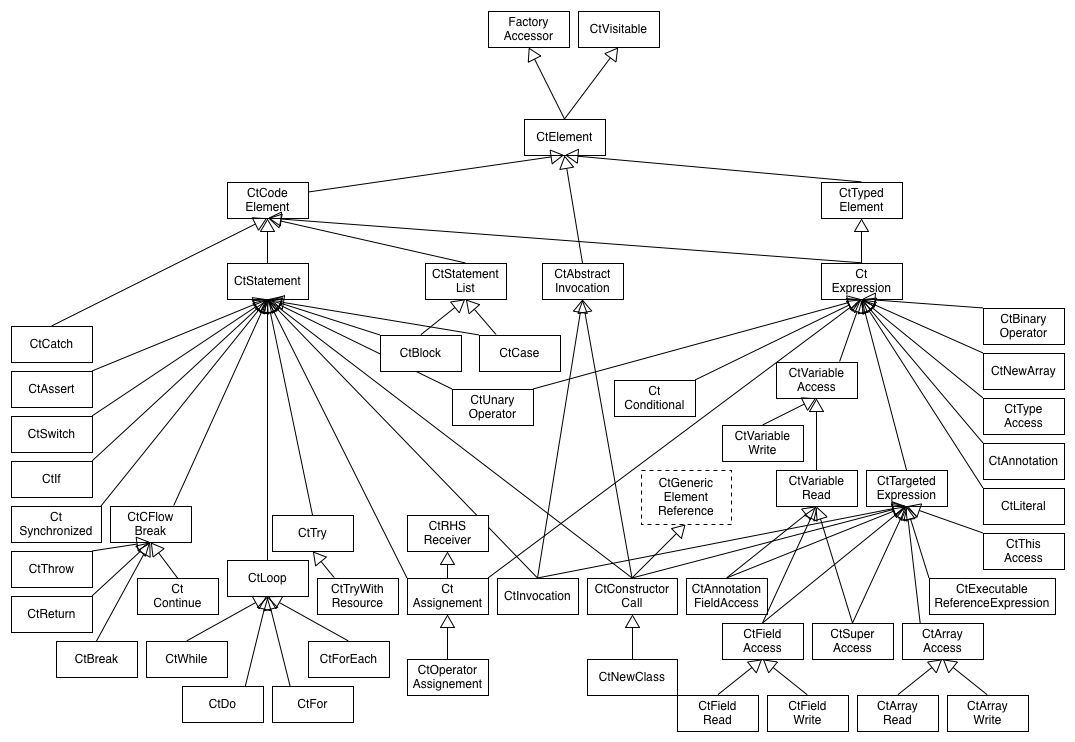
CtArrayRead
(javadoc)
int[] array = new int[10];
System.out.println(
array[0] // <-- array read
);
CtArrayWrite
(javadoc)
Object[] array = new Object[10];
// array write
array[0] = "new value";
CtAssert
(javadoc)
CtAssignment
(javadoc)
int x;
x = 4; // <-- an assignment
CtBinaryOperator
(javadoc)
// 3+4 is the binary expression
int x = 3 + 4;
CtBlock
(javadoc)
{ // <-- block start
System.out.println("foo");
}
CtBreak
(javadoc)
for(int i=0; i<10; i++) {
if (i>3) {
break; // <-- break statement
}
}
CtCase
(javadoc)
int x = 0;
switch(x) {
case 1: // <-- case statement
System.out.println("foo");
}
CtConditional
(javadoc)
System.out.println(
1==0 ? "foo" : "bar" // <-- ternary conditional
);
CtConstructorCall
(javadoc)
CtContinue
(javadoc)
for(int i=0; i<10; i++) {
if (i>3) {
continue; // <-- continue statement
}
}
CtDo
(javadoc)
int x = 0;
do {
x=x+1;
} while (x<10);
CtExecutableReferenceExpression
(javadoc)
java.util.function.Supplier p =
Object::new;
CtFieldRead
(javadoc)
class Foo { int field; }
Foo x = new Foo();
System.out.println(x.field);
CtFieldWrite
(javadoc)
class Foo { int field; }
Foo x = new Foo();
x.field = 0;
CtFor
(javadoc)
// a for statement
for(int i=0; i<10; i++) {
System.out.println("foo");
}
CtForEach
(javadoc)
java.util.List l = new java.util.ArrayList();
for(Object o : l) { // <-- foreach loop
System.out.println(o);
}
CtIf
(javadoc)
if (1==0) {
System.out.println("foo");
} else {
System.out.println("bar");
}
CtInvocation
(javadoc)
// invocation of method println
// the target is "System.out"
System.out.println("foo");
CtJavaDoc
(javadoc)
/**
* Description
* @tag a tag in the javadoc
*/
CtLambda
(javadoc)
java.util.List l = new java.util.ArrayList();
l.stream().map(
x -> { return x.toString(); } // a lambda
);
CtLiteral
(javadoc)
int x = 4; // 4 is a literal
CtLocalVariable
(javadoc)
// defines a local variable x
int x = 0;
// local variable in Java 10
var x = 0;
CtNewArray
(javadoc)
// inline creation of array content
int[] x = new int[] { 0, 1, 42}
CtNewClass
(javadoc)
// an anonymous class creation
Runnable r = new Runnable() {
@Override
public void run() {
System.out.println("foo");
}
};
CtOperatorAssignment
(javadoc)
int x = 0;
x *= 3; // <-- a CtOperatorAssignment
CtReturn
(javadoc)
Runnable r = new Runnable() {
@Override
public void run() {
return; // <-- CtReturn statement
}
};
CtSuperAccess
(javadoc)
class Foo { int foo() { return 42;}};
class Bar extends Foo {
int foo() {
return super.foo(); // <-- access to super
}
};
CtSwitch
(javadoc)
int x = 0;
switch(x) { // <-- switch statement
case 1:
System.out.println("foo");
}
CtSwitchExpression
(javadoc)
int i = 0;
int x = switch(i) { // <-- switch expression
case 1 -> 10;
case 2 -> 20;
default -> 30;
};
CtSynchronized
(javadoc)
java.util.List l = new java.util.ArrayList();
synchronized(l) {
System.out.println("foo");
}
CtTextBlock
(javadoc)
String example = """
Test String
""";
CtThisAccess
(javadoc)
class Foo {
int value = 42;
int foo() {
return this.value; // <-- access to this
}
};
CtThrow
(javadoc)
throw new RuntimeException("oops")
CtTry
(javadoc)
try {
System.out.println("foo");
} catch (Exception ignore) {}
CtTryWithResource
(javadoc)
// br is the resource
try (java.io.BufferedReader br = new java.io.BufferedReader(new java.io.FileReader("/foo"))) {
br.readLine();
}
CtTypeAccess
(javadoc)
// access to static field
java.io.PrintStream ps = System.out;
// call to static method
Class.forName("Foo")
// method reference
java.util.function.Supplier p =
Object::new;
// instanceof test
boolean x = new Object() instanceof Integer // Integer is represented as an access to type Integer
// fake field "class"
Class x = Number.class
CtTypePattern
(javadoc)
Object obj = null;
boolean longerThanTwo = false;
// String s is the type pattern, declaring a local variable
if (obj instanceof String s) {
longerThanTwo = s.length() > 2;
}
CtUnaryOperator
(javadoc)
int x=3;
--x; // <-- unary --
CtVariableRead
(javadoc)
String variable = "";
System.out.println(
variable // <-- a variable read
);
CtVariableWrite
(javadoc)
String variable = "";
variable = "new value"; // variable write
String variable = "";
variable += "";
CtWhile
(javadoc)
int x = 0;
while (x!=10) {
x=x+1;
};
CtYieldStatement
(javadoc)
int x = 0;
x = switch ("foo") {
default -> {
x=x+1;
yield x; //<--- yield statement
}
};
int x = 0;
x = switch ("foo") {
default -> 4; //<--- implicit yield statement
};
CtAnnotation
(javadoc)
// statement annotated by annotation @SuppressWarnings
@SuppressWarnings("unchecked")
java.util.List<?> x = new java.util.ArrayList<>()
CtClass
(javadoc)
// a class definition
class Foo {
int x;
}
CtInterface
(javadoc)
// an interface definition
interface Foo {
void bar();
}
CtRecord
(javadoc)
record Point(int x, int y) {
}